Permalink Manager provides a few useful API functions that could be used to create permalinks programatically.
If you want to integrate Permalink Manager with your own code, a third-party plugin, or an API, you have several options.
You can use the plugin to update the permalinks individually or in bulk directly from the admin panel. If you are only interested in learning how to change the permalink, please check out the corresponding article.
Updating Custom Permalinks
How to Get the Default Value of Custom Permalink?
If you just want Permalink Manager to store the custom permalink based on your default permalink formats, you must first generate it. Depending on the content type, you will need to use one of two following functions to generate the custom permalink based on your current permastructures settings.
As you can see below, only one argument is needed ($post or $term). You can use either the full object (instance of WP_Post() or WP_Term() class) or just its ID (as integer).
// A. [Posts, Pages, CPT items]
$new_uri = Permalink_Manager_URI_Functions_Post::get_default_post_uri($post);
// B. [Categories, Tags, custom taxonomy terms]
$new_uri = Permalink_Manager_URI_Functions_Tax::get_default_term_uri($term);
How to Save the Custom Permalink?
To save a custom permalink, you can use either the default value (see the previous section) or any text value. If the variable contains unallowed characters, the plugin will sanitize it inside Permalink_Manager_URI_Functions::save_single_uri() function.
Permalink_Manager_URI_Functions::save_single_uri($post_or_term_id, $new_uri, $is_term, $save_in_database);
The function requires four arguments:
Variable | Description |
---|---|
$post_or_term_id | The ID of the post/term whose permalink you want to change (integer) |
$new_uri | A new custom permalink that you want to use (string) |
$is_term | Set "true" if you want to update post permalinks, and "false" if you want to update terms (boolean) |
$save_in_database | If you wish to change the permalink in the database, set "true". (boolean) |
/**
* A. [Save Posts, Pages, CPT items permalinks]
*/
$post = ...
$post_id = ...
// A1. Generate the URI (optional)
$new_uri = Permalink_Manager_URI_Functions_Post::get_default_post_uri($post);
// A2. Save the URI in DB
Permalink_Manager_URI_Functions::save_single_uri($post_id, $new_uri, false, true);
/**
* B. [Save Categories, Tags, custom taxonomy terms permalinks]
*/
$term = ...
$term_id = ...
// B1. Generate the URI (optional)
$new_uri = Permalink_Manager_URI_Functions_Tax::get_default_term_uri($term);
// B2. Save the URI in DB
Permalink_Manager_URI_Functions::save_single_uri($term_id, $new_uri, true, true);
How to Execute PHP Code After Custom Permalink Update?
If necessary, you may execute some additional action everytime Permalink Manager updates the permalink. This could be useful, for example, if you want to immediately flush the CDN or cache.
function pm_custom_permalink_updated( $element_id, $new_uri, $old_uri ) {
	$current_action = current_filter();
	if ( $old_uri !== $new_uri ) {
		if ( $current_action === 'permalink_manager_updated_post_uri' ) {
			// Post ID: $element_id;
		} else if ( $current_action === 'permalink_manager_updated_term_uri' ) {
			// Term ID: $element_id;
		}
	}
}
add_action( 'permalink_manager_updated_post_uri', 'pm_custom_permalink_updated', 10, 3 );
add_action( 'permalink_manager_updated_term_uri', 'pm_custom_permalink_updated', 10, 3 );
Getting Custom Permalinks
The following two functions may be used to read the URI address assigned to a single post or term. If the custom permalink for this specific item was not previously stored in the custom permalinks array, Permalink Manager will provide the default URL as a fallback.
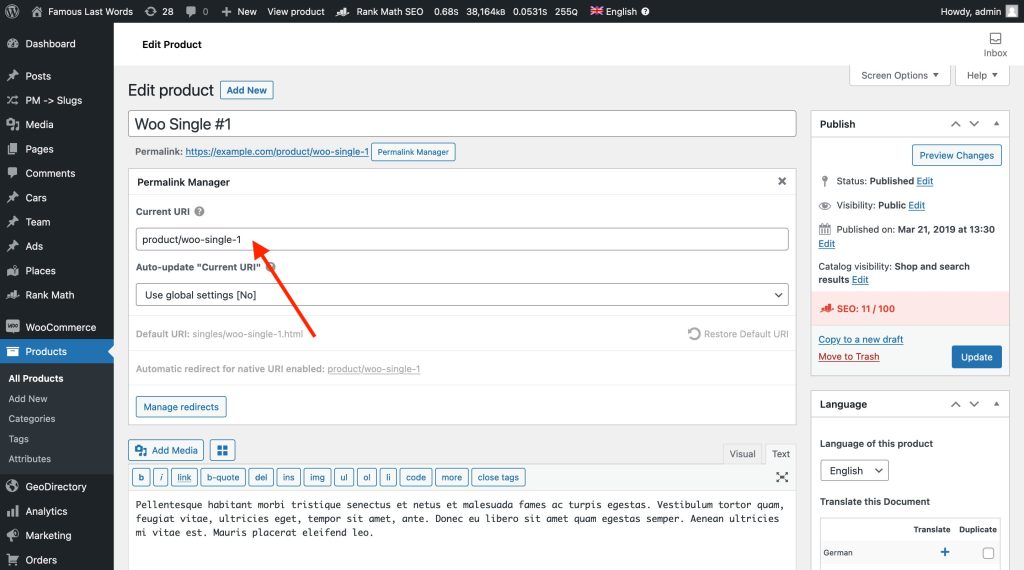
As with the previous function that returns the default URI, just one parameter ($post or $term) is required in this case. Both functions will accept either a full object (instance of the WP Post() or WP Term() classes) or its ID as a parameter (integer).
Posts, Pages and Custom Post Types
The above will just provide the URI, however you may obtain the complete URL address by using the WordPress inbuilt function get_permalink().
Permalink_Manager_URI_Functions_Post::get_post_uri($post)
Variable | Description |
---|---|
$post | The post ID or object (integer/WP_Post object) |
Categories, Tags and Custom Taxonomies
Permalink_Manager_URI_Functions_Tax::get_term_uri($term)
Variable | Description |
---|---|
$term | The term ID or object (integer/WP_Term object) |