You can find lots of resources about permalink plugins and how they help with SEO. However, WordPress offers a variety of other functions that can also be very useful.
While WordPress documentation explains these functions in detail, it can be hard to find them all in one place. To make it easier, I have gathered the most useful ones in the list below.
Please note that this list doesn’t include all available permalink functions. The ones listed are the most frequently used and essential for custom development.
How To Get WordPress Permalinks
Get single post/page permalink
get_permalink($post, $leavename);
Variable | Description |
---|---|
$post | Post identifier or object. If empty, the global $post variable will be used. (int|WP_Post) |
$leavename | If set true, the post/page name tag will be preserved. False by default (bool) |
If you work with plugins or themes, you will likely come across the
get_permalink()
function. It outputs the complete URL of any post, page, or custom post item.
One of the reasons this function is simple and effective. For example, if you need the full canonical URL of a post, all you need is its ID. Once you have it, the function will return the permalink as a string, which can then be used, e.g., directly inside a theme file.
$permalink = get_permalink(123);
echo '<a href="' . esc_url( $permalink ) . '">View post</a>';
In addition to the get_permalink(), an alias function called
get_the_permalink()
is also available. Both functions share the same set of variable inputs.
Alternatively, you may call specific functions such as
get_post_permalink()
for posts and custom post type items,
get_page_link()
for pages and
get_attachment_link()
for attachments.
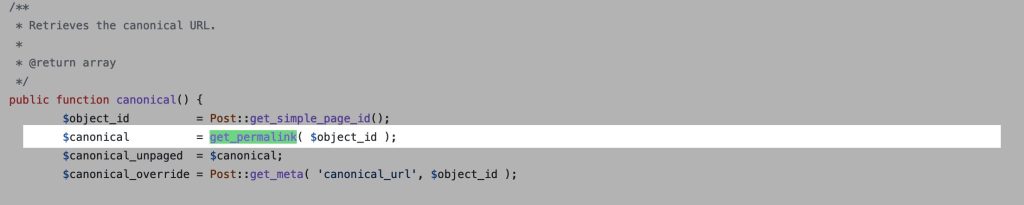
Get shortlink of post/page
wp_get_shortlink($post_id, $context = 'post', $allow_slugs = true);
Variable | Description |
---|---|
$post_id | Post identifier. If empty, the global $post->ID variable will be used. (int) |
$context | When this optional argument is set 'query' and function called on a single post page, the current query is used to determine the ID. (string) |
$allow_slugs | This optional argument is used only if the shortlink URL is modified with 'pre_get_shortlink' or 'get_shortlink' filters (bool) |
This function is similar to the
get_permalink()
function, but it returns a shorter URL that is intended to be more convenient for sharing.
The primary goal of
wp_get_shortlink()
function is to generate a shortlink URL that is limited by default to providing "?p=123" style links for posts. The
pre_get_shortlink
and
get_shortlink
filters in plugins may be used to change the shortlink format.
Get the ID of a post or page from its URL
If you need the ID of a post or page but only have its URL, the
url_to_postid()
function works the opposite of get_permalink(). All you need to do is provide the URL, and if it matches, the function will return the post or page's ID.
url_to_postid($post_url)
Variable | Description |
---|---|
$post_url | The full URL of the post or page (string) |
Using WordPress's
url_to_postid()
function is a fairly straightforward process. The function may be called straight from your WordPress template files or plugin code.
In the example below, we use the url_to_postid() function to get the post ID for the sample post URL. The
get_the_title()
function is then used to access the post title for that ID, which is then shown using the echo statement.
$url = 'https://www.example.com/sample-post/';
$post_id = url_to_postid( $url );
$post_title = get_the_title( $post_id );
echo $post_title;
Get single term permalink
get_term_link($post, $taxonomy);
Variable | Description |
---|---|
$term | The term object, ID, or slug for which a link needs to be found. (WP_Term|int|string) |
$taxonomy | The taxonomy of term provided in the first argument. (string) |
A term archive page is where all the posts related to a single term are shown together. Let’s say you have a taxonomy named "genre", and inside it, there is a term called "horror". The archive page for "horror" will display all the posts that fall under that category.
The
get_term_link()
method is similar to the
get_permalink()
function, except it returns the complete URL address of a single term.
Blog & WordPress archive link
The following is a list of three fundamental PHP functions that may be used to get the whole URL of the most frequently used archive links in WordPress. You may use them to lead your visitors to pages on your site that show all of the posts or items that belong to a custom post type.
Get post type archive URL
get_post_type_archive_link($post_type);
Variable | Description |
---|---|
$post_type | The name (slug) of the post type for which a URL to the archive page is to be found. (string) |
To display all items for a specific custom post type, such as all products or events, you need an archive page. This feature is built into WordPress and you can easily get the link to that page by using the
get_post_type_archive_link()
function with the post type name.
The function returns the archive link as a string, which you can then use to create a link to the post type archive page:
$archive_link = get_post_type_archive_link( 'product' );
echo '<a href="' . esc_url( $archive_link ) . '">View all products list</a>';
Author archive page URL
get_the_author_meta('user_url', $user_id);
Variable | Description |
---|---|
$field = 'user_url' | To acquire the archive URL, set the first parameter to 'user url'. (string) |
$user_id | The ID of the user whose archive URL is required. (integer) |
In contrast to the previously described functions, generating a URL for an author archive takes a little more finesse. To do this, use the
get_the_author_meta()
function and pass 'user url' as the first parameter.
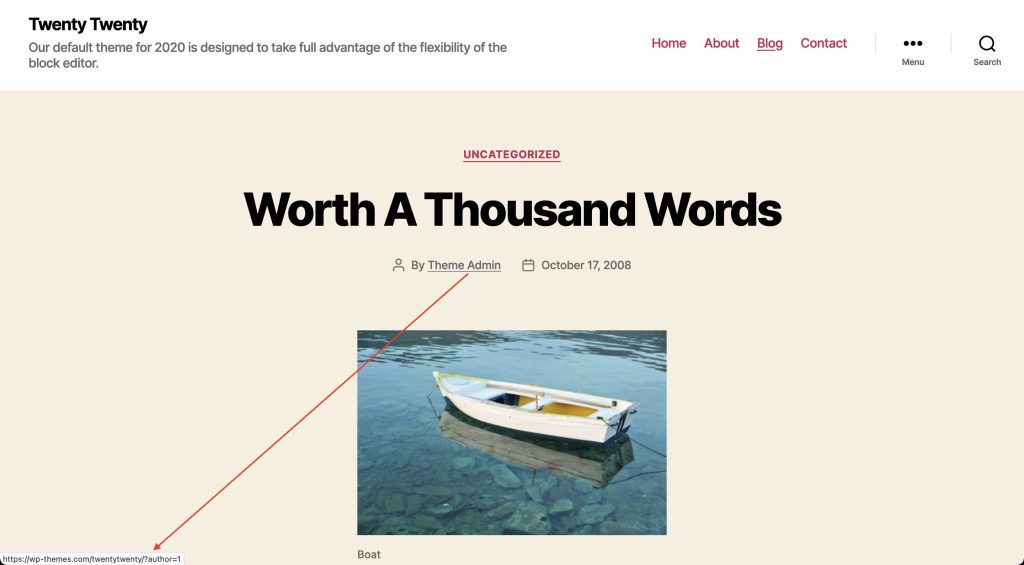
How to get blog page permalink in WordPress?
As you can see, each of the above-mentioned content kinds has its own dedicated function that returns the URL address. There are times when you need to provide the URL of the blog page where the posts are displayed.
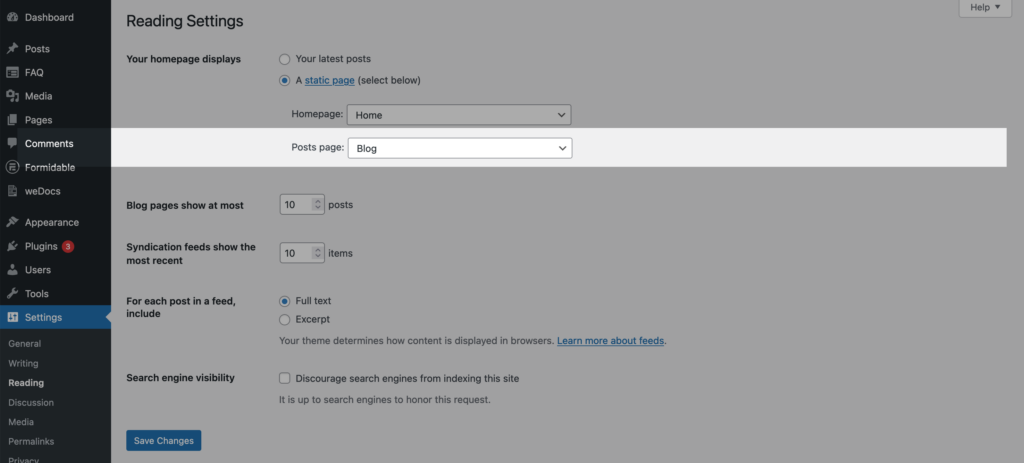
The code in the first line pulls the ID of the blog page selected under "Settings -> Reading" in the admin dashboard. It does this by calling the
get_option()
function, which fetches the ID from the database. In the second line, the
get_permalink()
function uses the fetched ID to return the canonical URL of the blog page as a string.
$blog_page_id = get_option('page_for_posts');
$blog_page_url = get_permalink($blog_page_id);
Even though it is not absolutely necessary, using the esc_url() function before showing a URL on a webpage is a good habit to prevent XSS attacks:
echo '<a href="' . esc_url($blog_page_url) . '">View blog</a>';
Homepage and template URLs
In most circumstances, get_home_url() and get_site_url() will return the same URL and may be used interchangeably. However, if you keep the WordPress source files in a separate folder, pay attention to the function you are using. If you are still confused, check out this StackOverflow discussion for a more in-depth explanation.
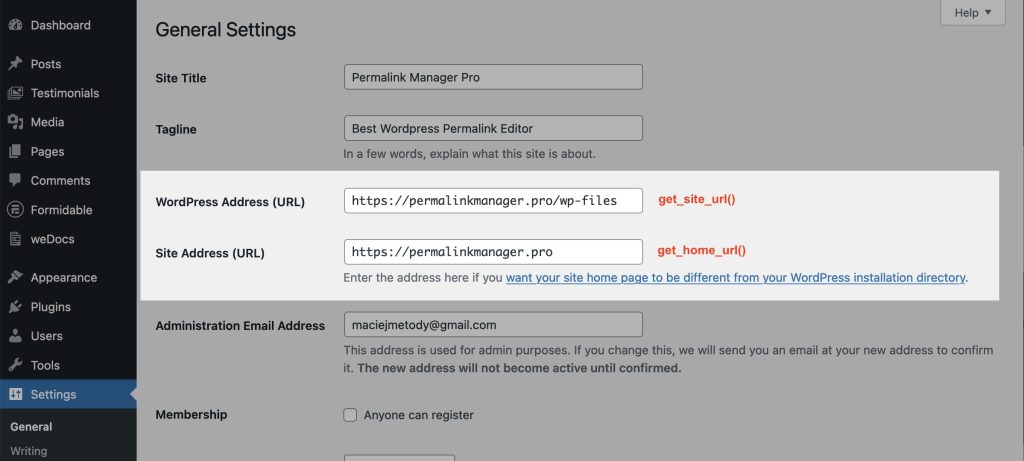
Homepage URL address
get_home_url($blog_id = null, $path = '', $scheme = null);
Variable | Description |
---|---|
$blog_id | If you are not using network/multisite configuration, leave this field empty. (integer) |
$path | Path relative to the home URL. (string) |
$scheme | Accepts 'http,' 'https', 'login', 'login post', 'admin', or 'relative' as parameters. (string) |
To get a homepage link please use the
get_home_url()
function. There is also a "shortcut" function available:
home_url()
. The function returns the value defined in the "Settings -> General" panel as the "Site Address". This function may come in handy if you want to provide a link to the front page in the navigation elements: breadcrumbs, sidebar or navbar.
WordPress files directory URL
get_site_url($blog_id = null, $path = '', $scheme = null);
Variable | Description |
---|---|
$blog_id | If you are not using network/multisite configuration, leave this field empty. (integer) |
$path | Path relative to the home URL. (string) |
$scheme | Accepts 'http,' 'https', 'login', 'login post', 'admin', or 'relative' as parameters. (string) |
This function returns the URL address of the directory where WordPress plugin files are stored. You can also use an alias function:
site_url()
. The function returns the address specified in the "Settings -> General" field in the "WordPress address (URL)" panel.
WordPress (child/parent) theme directory URL
get_stylesheet_directory_uri(); // Get child theme URL
get_template_directory_uri(); // Get parent theme URL
If you do not use a child theme,
get_stylesheet_directory_uri()
and
get_template_directory_uri()
will both return the same URL and may be used alternatively.
To put it another way, if you have a child theme installed, use
get_stylesheet_directory_uri()
to get the URL containing its directory. The
get_template_directory_uri()
function may be used to get the parent directory URL.
Both of these functions are useful for including resources (such as CSS or JavaScript files) in your theme. For example, you might use the
get_stylesheet_directory_uri()
function to link to a CSS file in your theme like this:
wp_enqueue_style( 'my-theme-style', get_stylesheet_directory_uri() . '/style.css' );
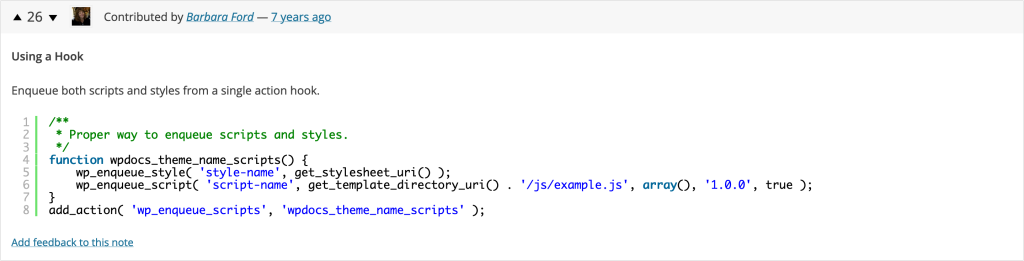
How to sanitize URL addresses?
Allowing unsanitized URLs in your code opens the door for attackers to insert harmful JavaScript into the URL. This could lead to security issues such as cross-site scripting (XSS) attacks, where an attacker is able to execute malicious code on your website.
esc_url($url, $protocols = '');
Variable | Description |
---|---|
$url | If you are not using network/multisite configuration, leave this field empty. (string) |
$protocols | Path relative to the home URL. (array of strings) |
You should always use
esc_url()
to sanitize URLs in HTML nodes (eg. <a href="">). The function will also reject URLs that do not use one of the whitelisted protocols: http, https, ftp, ftps, mailto, news, irc, gopher, nntp, feed, and telnet.
Invalid and potentially harmful characters will be automatically secured from input. For instance, ampersands (&) and single quotation marks (') will be encoded as numeric entity references (&, ').
Leave a Reply